Comparing JSON and XML Parsing in C#
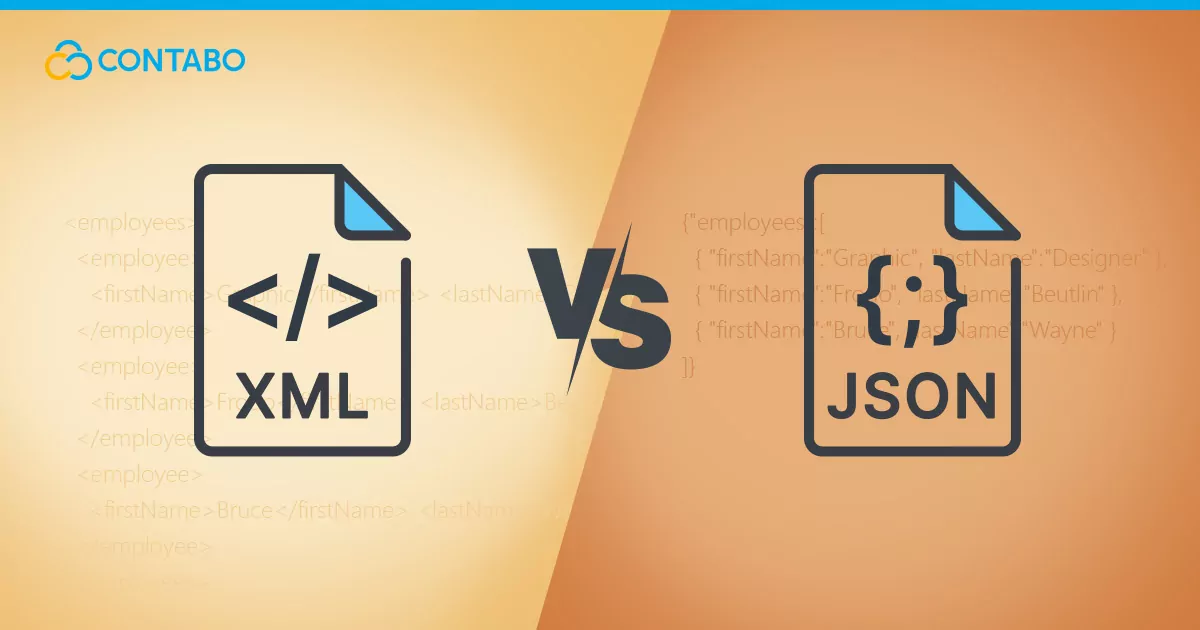
Converting Between JSON and XML in C#: A Deep Dive
Feature | JSON | XML |
---|---|---|
Readability | Easy | Complex |
Performance | Faster | Slower |
Storage Size | Small | Large |
Hierarchical Support | Limited | Strong |
Schema Validation | No | Yes |
XML and JSON are two of the most widely used data interchange formats in modern applications. While JSON has become increasingly popular due to its simplicity and lightweight nature, XML continues to be important in many enterprise systems and legacy applications. In this blog post, we’ll explore how to convert between these formats in C# applications, focusing on best practices and common pitfalls.
Understanding the Basics
Before diving into the conversion process, let’s briefly understand the structural differences between JSON and XML:
JSON (JavaScript Object Notation):
- Lightweight and easy to read
- Based on key-value pairs and arrays
- More concise than XML
- Native support in JavaScript
XML (eXtensible Markup Language):
- More verbose with opening and closing tags
- Supports attributes and namespaces
- Has a stricter structure
- Better support for complex hierarchical data
Setting Up Your Environment
To work with JSON and XML in C#, you’ll need these namespaces:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.Json;
using System.Xml.Linq;
Model Classes
First, let’s define some model classes that we’ll use for our conversions:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public List<Variant> Variants { get; set; }
public Discount Discount { get; set; }
}
public class Variant
{
public string Size { get; set; }
public decimal Weight { get; set; }
public decimal AdditionalCost { get; set; }
}
public class Discount
{
public string Type { get; set; }
public double Value { get; set; }
public DateTime StartDate { get; set; }
public DateTime ExpiryDate { get; set; }
public string Code { get; set; }
public bool IsActive { get; set; }
}
Converting XML to Object Model
Here’s how to parse XML into your object model:
private static List<Product> ParseProductsFromXml(string xmlString)
{
if (string.IsNullOrWhiteSpace(xmlString))
return new List<Product>();
try
{
var xDoc = XDocument.Parse(xmlString);
return xDoc.Root?
.Elements("Product")
.Select(x => new Product
{
Id = int.TryParse(x.Attribute("id")?.Value, out var id) ? id : 0,
Name = x.Attribute("name")?.Value ?? string.Empty,
Price = decimal.TryParse(x.Attribute("price")?.Value, out var price) ? price : 0,
Variants = x.Elements("Variant")
.Select(v => new Variant
{
Size = v.Attribute("size")?.Value ?? string.Empty,
Weight = decimal.TryParse(v.Attribute("weight")?.Value, out var weight) ? weight : 0,
AdditionalCost = decimal.TryParse(v.Attribute("additionalCost")?.Value, out var cost) ? cost : 0
}).ToList(),
Discount = new Discount
{
Type = x.Element("Discount")?.Attribute("type")?.Value ?? "None",
Value = double.TryParse(x.Element("Discount")?.Attribute("value")?.Value, out var value) ? value : 0,
StartDate = DateTime.TryParse(x.Element("Discount")?.Attribute("startDate")?.Value, out var startDate) ? startDate : DateTime.MinValue,
ExpiryDate = DateTime.TryParse(x.Element("Discount")?.Attribute("expiryDate")?.Value, out var expiryDate) ? expiryDate : DateTime.MinValue,
Code = x.Element("Discount")?.Attribute("code")?.Value ?? string.Empty,
IsActive = bool.TryParse(x.Element("Discount")?.Attribute("isActive")?.Value, out var isActive) && isActive
}
}).ToList() ?? new List<Product>();
}
catch (Exception ex)
{
Console.WriteLine($"XML Parsing Exception: {ex.Message}");
return new List<Product>();
}
}
Converting JSON to Object Model
Converting JSON to objects is more straightforward with System.Text.Json:
private static List<Product> ParseProductsFromJson(string jsonString)
{
if (string.IsNullOrWhiteSpace(jsonString))
return new List<Product>();
try
{
var options = new JsonSerializerOptions
{
PropertyNameCaseInsensitive = true
};
return JsonSerializer.Deserialize<List<Product>>(jsonString, options) ?? new List<Product>();
}
catch (Exception ex)
{
Console.WriteLine($"JSON Parsing Exception: {ex.Message}");
return new List<Product>();
}
}
Converting Object Model to XML
Here’s how to convert your object model back to XML:
private static string ConvertProductsToXml(List<Product> products)
{
if (products == null || !products.Any())
return "<Products></Products>";
var xDoc = new XElement("Products",
products.Select(p =>
{
var productElement = new XElement("Product",
new XAttribute("id", p.Id),
new XAttribute("name", p.Name),
new XAttribute("price", p.Price)
);
// Add variants
foreach (var variant in p.Variants ?? new List<Variant>())
{
productElement.Add(new XElement("Variant",
new XAttribute("size", variant.Size),
new XAttribute("weight", variant.Weight),
new XAttribute("additionalCost", variant.AdditionalCost)
));
}
// Add discount
productElement.Add(new XElement("Discount",
new XAttribute("type", p.Discount?.Type ?? "None"),
new XAttribute("value", p.Discount?.Value ?? 0),
new XAttribute("startDate", p.Discount?.StartDate.ToString("yyyy-MM-dd") ?? DateTime.MinValue.ToString("yyyy-MM-dd")),
new XAttribute("expiryDate", p.Discount?.ExpiryDate.ToString("yyyy-MM-dd") ?? DateTime.MinValue.ToString("yyyy-MM-dd")),
new XAttribute("code", p.Discount?.Code ?? string.Empty),
new XAttribute("isActive", (p.Discount?.IsActive ?? false).ToString().ToLower())
));
return productElement;
}));
return xDoc.ToString();
}
Converting Object Model to JSON
Converting objects to JSON is simpler:
private static string ConvertProductsToJson(List<Product> products)
{
if (products == null || !products.Any())
return "[]";
var options = new JsonSerializerOptions
{
WriteIndented = true
};
return JsonSerializer.Serialize(products, options);
}
Best Practices for JSON-XML Conversion
- Null Handling: Always check for null values and provide sensible defaults.
- Type Conversion: Use TryParse methods instead of direct casting to avoid exceptions.
- Error Handling: Implement robust error handling using try-catch blocks.
- Validation: Validate the input data before processing.
- Performance: Be mindful of performance implications when dealing with large datasets.
Common Pitfalls to Avoid
1. Ignoring XML Namespaces
XML namespaces can cause issues when parsing. When working with namespaced XML, use the proper namespace handling:
XNamespace ns = "http://example.com/namespace";
var elements = xDoc.Root?.Elements(ns + "Element");
2. Not Handling Special Characters
XML has special characters that need to be escaped. The XElement class handles this automatically, but be aware when manually constructing XML.
3. Forgetting Case Sensitivity
JSON property names are case-sensitive by default, while XML element names are also case-sensitive. Configure your serializer options appropriately:
var options = new JsonSerializerOptions
{
PropertyNameCaseInsensitive = true
};
4. Inefficient Parsing of Large Files
For large files, consider using streaming approaches:
using (var jsonDoc = JsonDocument.Parse(jsonString))
{
// Process document here
}
5. Not Handling Date Formats Correctly
Date formats can vary between XML and JSON. Always specify the date format explicitly:
p.Discount?.StartDate.ToString("yyyy-MM-dd") ?? DateTime.MinValue.ToString("yyyy-MM-dd")
Advanced Techniques
Custom Converters
For complex conversions, you can create custom JSON converters:
public class DateTimeConverter : JsonConverter<DateTime>
{
public override DateTime Read(ref Utf8JsonReader reader, Type typeToConvert, JsonSerializerOptions options)
{
return DateTime.Parse(reader.GetString());
}
public override void Write(Utf8JsonWriter writer, DateTime value, JsonSerializerOptions options)
{
writer.WriteStringValue(value.ToString("yyyy-MM-dd"));
}
}
Dynamic Conversion
For when you don’t know the structure in advance:
private static XElement JsonToXml(JsonElement element, string elementName)
{
var xElement = new XElement(elementName);
switch (element.ValueKind)
{
case JsonValueKind.Object:
foreach (var property in element.EnumerateObject())
{
xElement.Add(JsonToXml(property.Value, property.Name));
}
break;
case JsonValueKind.Array:
int index = 0;
foreach (var item in element.EnumerateArray())
{
xElement.Add(JsonToXml(item, $"Item{index++}"));
}
break;
default:
xElement.Value = element.ToString();
break;
}
return xElement;
}
Conclusion
Converting between JSON and XML in C# is a common task that requires careful handling of data structures and edge cases. By following the best practices outlined in this blog post and understanding the common pitfalls, you can ensure smooth conversion between these formats in your applications.
Remember that the choice between JSON and XML often depends on your specific use case, existing infrastructure, and integration requirements. Modern applications tend to favor JSON for its simplicity and reduced payload size, but XML continues to be important for integration with legacy systems and when complex document structures are needed.
I hope this guide helps you implement robust JSON-XML conversions in your C# applications!